Jul 6, 2006 at 10:22 PM
Join Date: Jun 18, 2006
Location: Montreal, Canada
Posts: 581
Age: 40
I've been toying around with NPCs lately and wanted to change the Mannan into a cannon of sorts. This would mean flipping its rect 90'. The NPC.tbl file, as has already been covered, doesn't permit this. I figured there may've been another table in the exe that handles this. After some poking around and hunting down pointers, I figured out how the game handles everything NPC-related.
Just like weapons, they're all handled through code. Sorry - NPC.tbl seems to be the most that a hex editor and no assembly knowhow will get you. Even the rendering bit is coded via assembly. For instance, the Mannan's frames are all RECTs MOVed into the stack byte by byte. Its code starts at 0x0002CCB0.
Once I finish my hack and begin releasing my info, I'll attempt to compile a list of each NPCs' relevant values when directly editable. As with weapons, there are a number of calculated values (particularly when an enemy can face multiple directions while being animated.) So no assembly knowhow can only get someone so far...
The pointer table SEEMS to start at 0x00098540. If you want to duplicate an enemy in the NPC.tbl file, you will have to duplicate its pointer (4 bytes each.) It should work, but I never tried it out.
Edit: Found some stuff out about how it makes an NPC spawn other NPCs (for instance, the Mannan firing out its projectile). First it pushes a large number of parameters on the stack (code simplified to save some space...)
push 0x0100 ; ?
push 0x00 ; ?
push [[ebp + 08] + 4c] ; ?
push 0x00 ; ?
push 0x00 ; ?
push XPOSITION
push YPOSITION
push NPC_ID
Then it makes a call to 0x0046efd0.
I saw that in the Heavy Press code when doing some research for caveoholic; it did the same with the butes. I'm still not clear on some parameters though. I suspect the 3rd one is the direction...
There's a function table for Mannan at 0x0002cffd. Screwing with it was a fun experience...
It won't stop exploding and showering me with items!!
Edit: Little correction. That's 0x00098548, not 0x00098540.
Just like weapons, they're all handled through code. Sorry - NPC.tbl seems to be the most that a hex editor and no assembly knowhow will get you. Even the rendering bit is coded via assembly. For instance, the Mannan's frames are all RECTs MOVed into the stack byte by byte. Its code starts at 0x0002CCB0.
Once I finish my hack and begin releasing my info, I'll attempt to compile a list of each NPCs' relevant values when directly editable. As with weapons, there are a number of calculated values (particularly when an enemy can face multiple directions while being animated.) So no assembly knowhow can only get someone so far...
The pointer table SEEMS to start at 0x00098540. If you want to duplicate an enemy in the NPC.tbl file, you will have to duplicate its pointer (4 bytes each.) It should work, but I never tried it out.
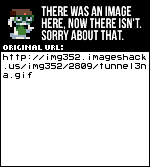
Edit: Found some stuff out about how it makes an NPC spawn other NPCs (for instance, the Mannan firing out its projectile). First it pushes a large number of parameters on the stack (code simplified to save some space...)
push 0x0100 ; ?
push 0x00 ; ?
push [[ebp + 08] + 4c] ; ?
push 0x00 ; ?
push 0x00 ; ?
push XPOSITION
push YPOSITION
push NPC_ID
Then it makes a call to 0x0046efd0.
I saw that in the Heavy Press code when doing some research for caveoholic; it did the same with the butes. I'm still not clear on some parameters though. I suspect the 3rd one is the direction...
There's a function table for Mannan at 0x0002cffd. Screwing with it was a fun experience...
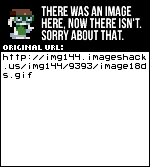
It won't stop exploding and showering me with items!!

Edit: Little correction. That's 0x00098548, not 0x00098540.