Aug 19, 2016 at 6:58 AM
Join Date: Aug 28, 2009
Location: The Purple Zone
Posts: 5998
Pronouns: he/him
Hey there
As you may or may not know, GUXT is the top-down shoot them up game made by Pixel in 2007. So far, to my knowledge, nobody has ever managed to mod it. But I don't think it should be too hard - all the data files are right there, in Pixel's signature style. So I might pick it apart and try to document what I find
First off is .pximg files. Now,at this point Pixel is still obfuscating his data but he's got some new tricks up his sleeve. Trying to open the image as a bitmap reveals a scrambled mess! Each row in the scrambled image can be passed through a hashing function to give its position in the real image. I've included a java implementation of the function below. Forgive the clumsiness at parts but Java doesn't play well with unsigned types.
The output of this gives the lines of the scrambled image in the order they appear in the unscrambled image. e.g.
[9, 6, 5, 12, 11, 7, 4, 10, 2, 13, 14, 3, 0, 1, 15, 8]
so the 0th row in the unscrambled image is the 9th row in the scrambled one.
Now with that I've had access to all of Guxt's image assets, and one file in particular stands out to me
I believe these are the icons used in pixel's own stage editor. Conveniently, they're probably also in the order of each unit's Entity ID. So this should help decode those a lot.
As you may or may not know, GUXT is the top-down shoot them up game made by Pixel in 2007. So far, to my knowledge, nobody has ever managed to mod it. But I don't think it should be too hard - all the data files are right there, in Pixel's signature style. So I might pick it apart and try to document what I find
First off is .pximg files. Now,at this point Pixel is still obfuscating his data but he's got some new tricks up his sleeve. Trying to open the image as a bitmap reveals a scrambled mess! Each row in the scrambled image can be passed through a hashing function to give its position in the real image. I've included a java implementation of the function below. Forgive the clumsiness at parts but Java doesn't play well with unsigned types.
Code:
public class Decoder {
int img_height;
int[] line_indexes;
int hash_a;
int hash_b;
public Decoder(int h, int a, int b) {
hash_a = a;
hash_b = b;
img_height = h;
line_indexes = new int[img_height];
Arrays.fill(line_indexes, -1);
int current_line = 0;
int cycle_count;
for (int i = 0; i < img_height; i++) {
cycle_count = (shuffle() >> 8) & 0xFF;
if (cycle_count == 0) {
cycle_count = 1;
}
do {
current_line = (current_line + 1) % img_height;
if (line_indexes[current_line] == -1)
--cycle_count;
} while (cycle_count != 0);
line_indexes[current_line] = i;
}
System.out.println(Arrays.toString(line_indexes));
}
private int shuffle() {
int tmp = (hash_a + hash_b) & 0xFFFF;
byte low = (byte) tmp;
byte high = (byte) (tmp >>> 8);
short result = (short) (low << 8);
result |= high & 0xFF;
hash_b = hash_a;
hash_a = result;
return result;
}
public static void main(String[] args) {
new Decoder(16, 0x4444, 0x8888);
}
}
The output of this gives the lines of the scrambled image in the order they appear in the unscrambled image. e.g.
[9, 6, 5, 12, 11, 7, 4, 10, 2, 13, 14, 3, 0, 1, 15, 8]
so the 0th row in the unscrambled image is the 9th row in the scrambled one.
Now with that I've had access to all of Guxt's image assets, and one file in particular stands out to me
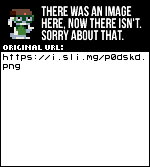
I believe these are the icons used in pixel's own stage editor. Conveniently, they're probably also in the order of each unit's Entity ID. So this should help decode those a lot.